In this blog I am trying to explain the concept of Convert Data Table to XML, XSD, and HTML.
This involve following steps as:
Step1:Let’s create a data table and add some rows and column on it.
DataTable dt = new DataTable()
{
TableName = "employee"
};
DataColumn keyColumn = dt.Columns.Add("ID", typeof(System.Int32)); dt.Columns.Add("Name", typeof(System.String)); dt.Columns.Add("Desig", typeof(System.String)); dt.Columns.Add("Address", typeof(System.String)); dt.Columns.Add("MobileNo", typeof(System.String)); dt.Columns.Add("gender", typeof(System.String)); dt.PrimaryKey = new DataColumn[] { keyColumn }; dt.Rows.Add(new object[] { 101," Ashish","programmer","25369874125","male"}); dt.Rows.Add(new object[] { 102, " Shubham", "tester","2536568923","male"}); dt.Rows.Add(new object[] { 103, " Anupam", "developer","9807167825","male"}); dt.Rows.Add(new object[] { 104, " Anurag", "Sysanalyst","945632012","male"}); dt.AcceptChanges();
Step2: Now convert data table into xml
using (TextWriter writer = new StringWriter()) { dt.WriteXml(writer);
var xml = writer.ToString();
Console.WriteLine("-----------------xml format----------------------"); Console.WriteLine(xml);
// following lines convert xml into html
}
At this point of time you have successfully created the data table and convertint it into xml file.
Now in order to convert xml data in to html we need to know basics of XSLT. Introduction to XSLT:
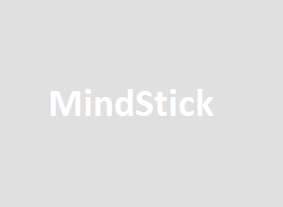
XSLT is capable of programming as looping, branching with IF and
declaring variable etc. In other words XSLT allows you to define variables,
you can have loops, you can have condition checks, and many more...
So now it is the time to start to create XSLT file in your program.
Step4: Right click on your project in solution explorer and a XSLT file, give
name it as “Sample.xslt” and the default code will be display as follows:
<?xml version="1.0" encoding="utf-8"?>
<xsl:stylesheet version="1.0" xmlns:xsl="http://www.w3.org/1999/XSL/Transform" xmlns:msxsl="urn:schemas-microsoft-com:xslt" exclude-result-prefixes="msxsl"> <xsl:output method="xml" indent="yes"/>
<xsl:template match="@* | node()">
<xsl:copy>
<xsl:apply-templates select="@* | node()"/>
</xsl:copy>
</xsl:template>
</xsl:stylesheet>
Step5: Add your codes in XSLT file as given
<?xml version="1.0" encoding="utf-8"?>
<xsl:stylesheet version="1.0" xmlns:xsl="http://www.w3.org/1999/XSL/Transform" xmlns:msxsl="urn:schemas-microsoft-com:xslt" exclude-result-prefixes="msxsl"> <xsl:output method="html" omit-xml-declaration="yes" indent="yes"/> <xsl:template match="@* | node()"> <html>
<body>
<table>
<tr>
<xsl:for-each select="/*/node()">
<xsl:if test="position()=1">
<xsl:for-each select="*">
<td>
<xsl:value-of select="local-name()"/>
</td>
</xsl:for-each>
</xsl:if>
</xsl:for-each>
</tr>
<xsl:for-each select="*">
<tr>
<xsl:for-each select="*">
<td>
<xsl:value-of select="."/>
</td>
</xsl:for-each>
</tr>
</xsl:for-each>
</table>
</body>
</html>
</xsl:template>
</xsl:stylesheet>
Step6: Add a class in your project say”xltOperation.cs” and add code as follows
using System.Xml.Linq;
using System.Xml;
using System.Xml.Xsl;
namespace DatatabletoXmlConversition
{
class XltOperation
{
public string GetValue(string templatePath, string xmlString)
{
XDocument xmlObj = XDocument.Parse(xmlString);
XDocument result = GetResultXml(templatePath, xmlObj);
return(result==null)?string.Empty:result.Document.ToString();
}
private XDocument GetResultXml(string templatePath, XDocument xmlObj)
{
XDocument result = new XDocument();
using (XmlWriter writer = result.CreateWriter())
{ XslCompiledTransform xslt = new XslCompiledTransform();
xslt.Load(templatePath);
xslt.Transform(xmlObj.CreateReader(), writer);
}
return result;
}
}
}
The XslCompiledTransform class in the System.Xml.Xsl namespace. This class transforms XML data using an XSLT stylesheet. We just have to use two methods of this class: Load and Transform. The Load () method loads the defined XSLT file into XslCompiledTransformed class object and method Transform () actually transform the XML file into output file.
Step6: Finally build and run your project. You will got output as following screen shows.
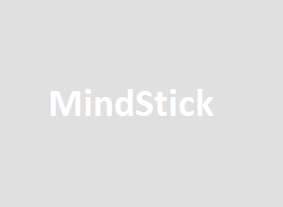
And HTML output in below the XML output as
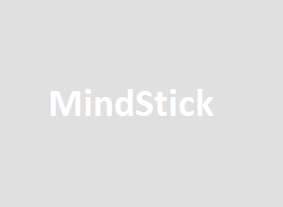
Leave Comment