In this blog I am trying to explain the concept of Convert Class in C#.
Convert Class
Convert class returns a type whose value is equivalent to the value of a specified type. The supported base types are Boolean, Char, SByte, Byte, Int16, Int32, Int64, UInt16, UInt32, UInt64, Single, Double, Decimal, DateTime and String.
A conversion method exists to convert every base type to every other base type. However, the actual call to a particular conversion method can produce one of four outcomes, depending on the value of the base type at run time and the target base type. These four outcomes are:
· No conversion. This occurs when an attempt is made to convert from a type to itself (for example, by calling Convert.ToInt32(Int32) with an argument of type Int32). In this case, the method simply returns an instance of the original type.
· An InvalidCastException. This occurs when a particular conversion is not supported. An InvalidCastException is thrown for the following conversions:
o Conversions from Char to Boolean, Single, Double, Decimal, or DateTime.
o Conversions from Boolean, Single, Double, Decimal, or DateTime to Char.
o Conversions from DateTime to any other type except String.
o Conversions from any other type, except String, to DateTime.
· A successful conversion. For conversions between two different base types not listed in the previous outcomes, all widening conversions as well as all narrowing conversions that do not result in a loss of data will succeed and the method will return a value of the targeted base type.
· An OverflowException. This occurs when a narrowing conversion results in a loss of data. For example, trying to convert a Int32 instance whose value is 10000 to a Byte type throws an OverflowException because 10000 is outside the range of the Byte data type.
Code Example
// Sample for the Convert class summary.
using System;
class ConvertorClass
{
public static void Main()
{
bool xBool = true;
short xShort = 1;
int xInt = 2;
long xLong = 3;
float xSingle = 4.0f;
double xDouble = 5.0;
decimal xDecimal = 6.0m;
string xString = "7";
char xChar = '8'; // '8' = hexadecimal 38 = decimal 56
byte xByte = 9;
// The following types are not CLS-compliant.
ushort xUshort = 120;
uint xUint = 121;
ulong xUlong = 122;
sbyte xSbyte = 123;
Console.WriteLine("Boolean: {0}", Convert.ToInt64(xBool));
Console.WriteLine("Int16: {0}", Convert.ToInt64(xShort));
Console.WriteLine("Int32: {0}", Convert.ToInt64(xInt));
Console.WriteLine("Int64: {0}", Convert.ToInt64(xLong));
Console.WriteLine("Single: {0}", Convert.ToInt64(xSingle));
Console.WriteLine("Double: {0}", Convert.ToInt64(xDouble));
Console.WriteLine("Decimal: {0}", Convert.ToInt64(xDecimal));
Console.WriteLine("String: {0}", Convert.ToInt64(xString));
Console.WriteLine("Char: {0}", Convert.ToInt64(xChar));
Console.WriteLine("Byte: {0}", Convert.ToInt64(xByte));
Console.WriteLine("DateTime: There is no example of this conversion because");
Console.WriteLine(" a DateTime cannot be converted to an Int64.");
Console.WriteLine("The following types are not CLS-compliant.\n");
Console.WriteLine("UInt16: {0}", Convert.ToInt64(xUshort));
Console.WriteLine("UInt32: {0}", Convert.ToInt64(xUint));
Console.WriteLine("UInt64: {0}", Convert.ToInt64(xUlong));
Console.WriteLine("SByte: {0}", Convert.ToInt64(xSbyte));
Console.ReadKey();
}
}
Note: - The DateTime type cannot be converted to an Int64. (DateTime
xDateTime = DateTime.Now;)
Code description:
1. Using System (include the System namespace).
2. Create a Convertor class.
4. Start Mani method.
6. to 20. Create all types of variable with its initial value.
21. to 37. Show all variable value with covert into ToInt64(means integer
vale with 64 bit) .
Output:-
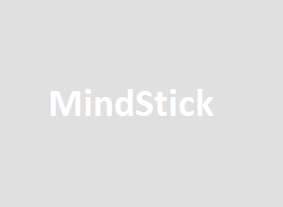
Leave Comment