In this blog, I’m trying to explain the concept of timer in .net
The Timer component is a server-based timer, which allows you to specify a recurring interval at which the Elapsed event is raised in your application. You can then handle this event to provide regular processing. For example, suppose you have a critical server that must be kept running 24 hours a day, 7 days a week. You could create a service that uses a Timer to periodically check the server and ensure that the system is up and running. If the system is not responding, the service could attempt to restart the server or notify an administrator.
The Timer component raises the Elapsed event, based on the value of the Interval property. You can handle this event to perform the processing you need. For example, suppose that you have an online sales application that continuously posts sales orders to a database. The service that compiles the instructions for shipping operates on a batch of orders rather than processing each order individually. You could use a Timer to start the batch processing every 30 minutes.
Example
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Timers;
namespace TimerConsoleApplication2
{
class Program
{
static void Main(string[] args)
{
System.Timers.Timer atimer = new System.Timers.Timer();
atimer.Elapsed += new ElapsedEventHandler(OnTimedEvent);
// Set the Interval to 5 seconds.
atimer.Interval = 5000;
atimer.Enabled = true;
Console.WriteLine("Press \'q\' to quit the sample.");
while (Console.Read() != 'q') ;
}
// Specify what you want to happen when the Elapsed event is raised.
private static void OnTimedEvent(object source, ElapsedEventArgs e)
{
Console.WriteLine("Hello World!");
}
}
}
Output
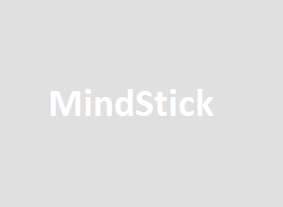
Leave Comment