Delegate Class in C#
In this blog I am trying to explain the concept of Delegate Class in C#.
Delegate Class
It is represents a delegate (representative), which is a data structure that refers to a static method or to a class instance and an instance method of that class.
The Delegate class is the base class for delegate types. However, only the system and compilers can derive explicitly from the Delegate class or from the MulticastDelegate class. It is also not permissible to derive a new type from a delegate type. The Delegate class is not considered a delegate type; it is a class used to derive delegate types.
Example: -
1. using System;
2. publicclassMindstickSamplesDelegate
3. {
4. publicdelegateStringmyMethodDelegate(int myInt);
5. publicclassmySampleClass
6. {
7. publicString myStringMethod(int myInt)
8. {
9. if (myInt > 0)
10.return ("positive");
11.if (myInt < 0)
12.return ("negative");
13.return ("zero");
14.}
15.publicstaticString mySignMethod(int myInt)
16.{
17.if (myInt > 0)
18.return ("+");
19.if (myInt < 0)
20.return ("-");
21.return ("");
22.}
23.}
24.publicstaticvoid Main()
25.{
26.mySampleClass mySC = newmySampleClass();
27.myMethodDelegate myD1 = newmyMethodDelegate(mySC.myStringMethod);
28.myMethodDelegate myD2 = newmyMethodDelegate(mySampleClass.mySignMethod);
29.Console.WriteLine("{0} is {1}; use the sign \"{2}\".", 5, myD1(5), myD2(5));
30.Console.WriteLine("{0} is {1}; use the sign \"{2}\".", -3, myD1(-3), myD2(-3));
31.Console.WriteLine("{0} is {1}; use the sign \"{2}\".", 0, myD1(0), myD2(0));
32.Console.ReadKey();
33.}
34.}
Code Description: -
1. Using System (include the System namespace).
2. Create MindstickSamplesDelegate class.
4. Declares a delegate for a method that takes in an int and returns a String.
5. Defines some methods to which the delegate can point.
7. Defines an instance method.
15. Defines a static method.
24. Create main method.
26. to 28. Creates one delegate for each method. For the instance method, an instance (mySC) must be supplied. For the static method, use the class name.
29. to 31. Invokes the delegates.
Output: -
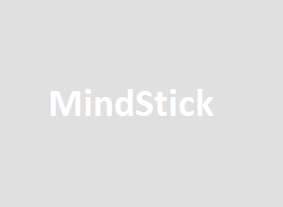
Anonymous User
22-Jul-2019Thanks for sharing.